Suggestions on a design pattern for logging in .NET?
Hello! I’m starting a personal project in .NET.
For a logging solution, I want to achieve something similar to what I have in a Python REST API I wrote a while back using the decorator pattern (example in the image).
In the example, the outter “log” function receives the logger I want to use, the decorator function receives a function and returns the decorated function ready to be called (this would be the decorator), and the wrapper function receives the same arguments as the function to be decorated (which is a generic (*args, **kwargs) ofc, because it’s meant to decorate any function) and returns whatever the return type of the function is (so, Any). In lines 17 - 24 I just call the passed in “func” with the passed in arguments, but in between I wrap it in a try except block and log that the function with name func.__name__
started or finished executing. In practice, using this decorator in Python looks like this:
<span style="color:#323232;">import logging
</span><span style="color:#323232;">from my.decorator.module import log
</span><span style="color:#323232;">
</span><span style="color:#323232;">_logger = logging.getLogger(__name__)
</span><span style="color:#323232;">
</span><span style="color:#323232;">@log(_logger)
</span><span style="color:#323232;">def my_func(arg1: Arg1Type, arg2: Arg2Type) -> ReturnType:
</span><span style="color:#323232;"> ...
</span>
Ofc it’s a lot simpler in Python, however I was wondering if it would be possible or even recommended to attempt something similar in C#. I wouldn’t mind having to call the function and wrap it manually, something like this:
return RunWithLogs(MyFunction, arg1, arg2);
What I do want to avoid is manually writing the log statements inside the service’s business logic, or having to write separate wrappers for each method I want to log. Would be nice to have one generic function or class that I can somehow plug-in to any method and have it log when the call starts and finishes.
Any suggestions? Thanks in advance.
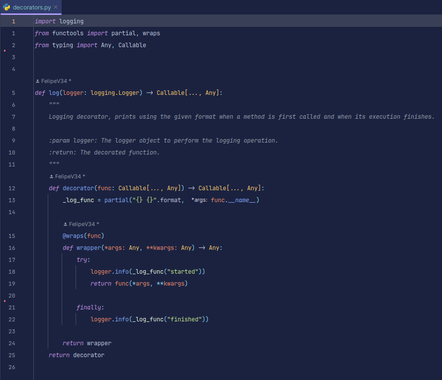
Add comment