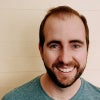
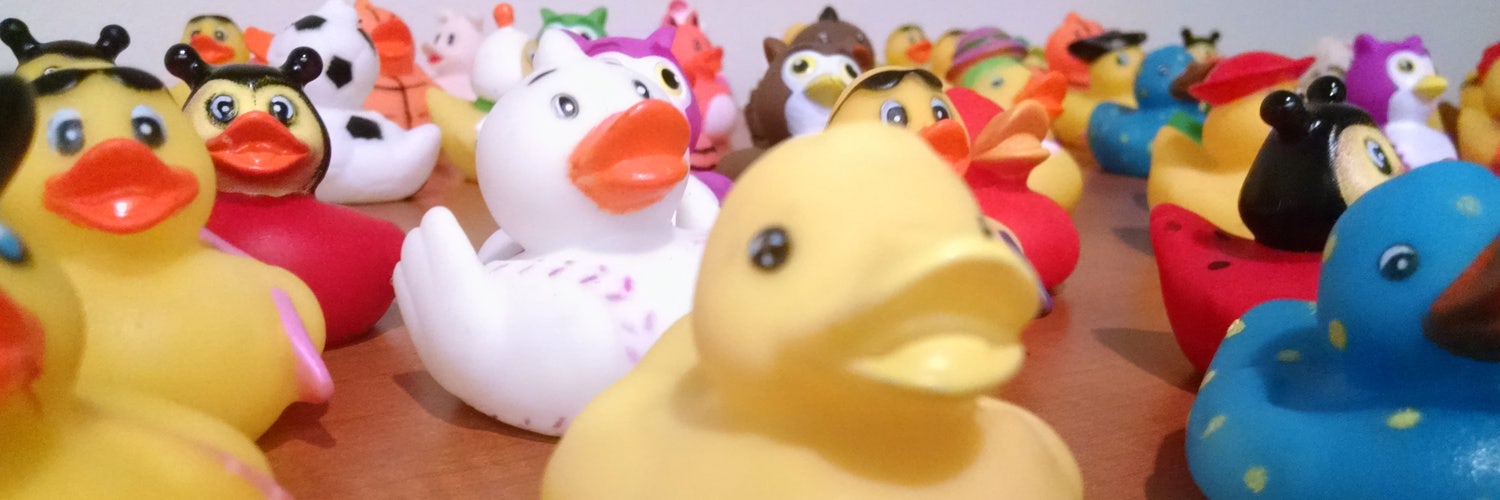
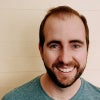
#Python & #Django educator & team trainer
I help folks sharpen their Python skills with https://PythonMorsels.com🐍🍪
Also a #humanist #YIMBY who is attempting more ethical eating (#vegetarian, not yet #vegan) and thinks #economics is highly underrated, but I don't post about those topics very often.
he/him
This profile is from a federated server and may be incomplete. Browse more on the original instance.