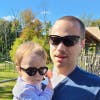
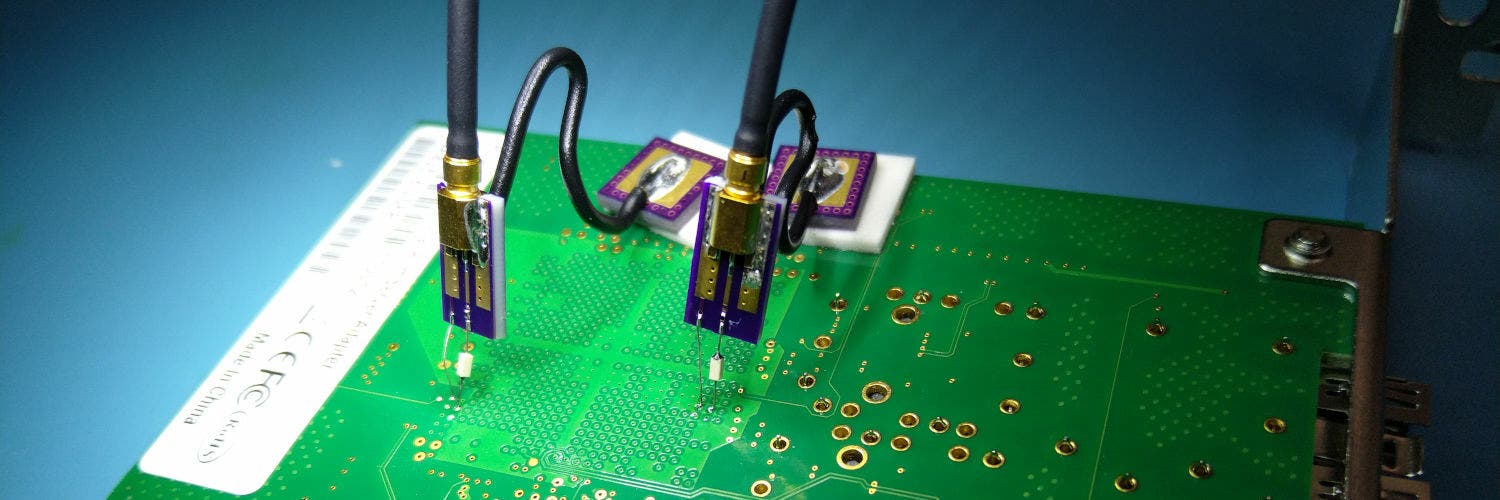
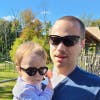
Security and open source at the hardware/software interface. Embedded sec @ IOActive. Lead dev of ngscopeclient/libscopehal. GHz probe designer. Open source networking hardware. "So others may live"
Toots searchable on tootfinder.
This profile is from a federated server and may be incomplete. Browse more on the original instance.