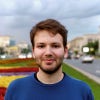
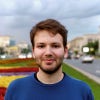
Unix hacker. I do obscure and cursed things.
I hack on Darling, SerenityOS / Ladybird, GNU Hurd / glibc, wl-clipboard, Owl, etc.
I use GNOME, and contribute to freedesktop / GNOME projects sometimes (systemd, PipeWire, GLib, GTK, etc).
I like Rust and dislike Docker.
This profile is from a federated server and may be incomplete. Browse more on the original instance.
Private