When to Use Arc Instead of Vec
Rust lets you do efficient reference-counted strings and dynamic arrays using Arc basically just as easily as their owning (and deep-cloning) equivalents, String and Vec respectively. So why not use them as a reasonable default, until you actually need the mutability that String and Vec provide? Get into the weeds with me here, feat. some cool visualizations, with special guest appearance from Box.
This video assumes some familiarity with Rust and its core smart pointer types, namely Vec/String/Rc/Arc/Box, along with data structures like HashMap and BTreeMap, and traits like Clone, Hash, Ord, and serde::{Serialize, Deserialize}.
serde feature flag for Rc/Arc
Arc docs
Vec docs
Smart pointers in Rust • Crust of Rust
animations
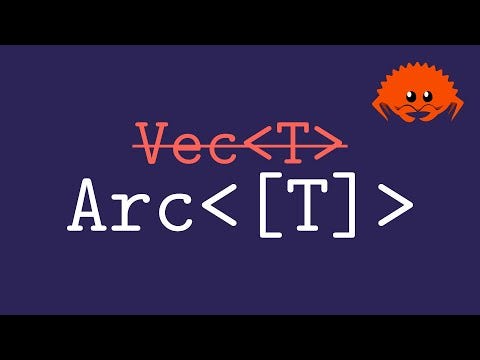
Add comment